This Getting Started guide walks you through the process of building an application that uses Spring for Android's
RestTemplate
to consume a Spring MVC-based RESTful web service.Compile và chạy ứng dụng rest server
You will build an Android client that consumes a Spring-based RESTful web service. Specifically, the client will consume the service created in Building a RESTful Web Servce.
Chạy spring boot rest: java -jar gs-rest-service-0.1.0.jar
The Android client will be accessed through an Android emulator, and will consume the service accepting requests at:
http://192.168.1.3:8080/greeting
The service will respond with a JSON representation of a greeting:
{"id":1,"content":"Hello, World!"}
Bạn sẽ cần những gì
- Thời gian khoảng 15 phút
- Android Studio
- Labtop có kết nối internet
Tạo Android project và cập nhật mã nguồn client
Source code hoàn chỉnh ở URL = https://github.com/spring-guides/deprecate-gs-consuming-rest-android/tree/master/complete
Within Android Studio, create a new project. If you prefer, you can use the project in the
initial
folder and skip ahead to Create a representation class. When you are finished, you can compare your code to the complete
folder and Run the client. Use "Rest" for the application and module names, and modify the package name to be "org.hello.rest". Enter the location of your choosing for the project and leave all the other options with their default settings.
The next screen presents some options for configuring the app icons. Continue with the default options.
To complete this guide, you will edit the following:
- Rest/src/main/AndroidManifest.xml
- Rest/src/main/res/values/strings.xml
- Rest/src/main/res/layout/fragment_main.xml
- Rest/src/main/res/menu/main.xml
- Rest/build.gradle
- Rest/src/main/java/org/hello/rest/MainActivity.java
Lưu ý những file sau:
build.gradle
apply plugin: 'com.android.application'
android {
compileSdkVersion 23
buildToolsVersion "26.0.1"
defaultConfig {
applicationId "org.hello.rest"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'),
'proguard-rules.txt'
'proguard-rules.txt'
}
}
packagingOptions {
exclude 'META-INF/DEPENDENCIES.txt'
exclude 'META-INF/LICENSE.txt'
exclude 'META-INF/NOTICE.txt'
exclude 'META-INF/NOTICE'
exclude 'META-INF/LICENSE'
exclude 'META-INF/DEPENDENCIES'
exclude 'META-INF/notice.txt'
exclude 'META-INF/license.txt'
exclude 'META-INF/dependencies.txt'
exclude 'META-INF/LGPL2.1'
}
}
dependencies {
compile 'com.android.support:appcompat-v7:23.0.0'
compile fileTree(dir: 'libs', include: ['*.jar'])
compile
'org.springframework.android:spring-android-rest-template:1.0.1.RELEASE'
'org.springframework.android:spring-android-rest-template:1.0.1.RELEASE'
compile 'com.fasterxml.jackson.core:jackson-databind:2.3.2'
compile 'joda-time:joda-time:2.9.4'
}
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.hello.rest"
android:versionCode="1"
android:versionName="1.0.0" >
<uses-sdk
android:minSdkVersion="19"
android:targetSdkVersion="19" />
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme">
<activity
android:name="org.hello.rest.MainActivity"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
MainActivity.java
package org.hello.rest;
import android.os.AsyncTask;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v7.app.ActionBarActivity;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import org.springframework.http.converter.json.
MappingJackson2HttpMessageConverter;
MappingJackson2HttpMessageConverter;
import org.springframework.web.client.RestTemplate;
import org.hello.rest.Greeting;
public class MainActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (savedInstanceState == null) {
getSupportFragmentManager().beginTransaction()
.add(R.id.container, new PlaceholderFragment())
.commit();
}
}
@Override
protected void onStart() {
super.onStart();
new HttpRequestTask().execute();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_refresh) {
new HttpRequestTask().execute();
return true;
}
return super.onOptionsItemSelected(item);
}
/**
* A placeholder fragment containing a simple view.
*/
public static class PlaceholderFragment extends Fragment {
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(
R.layout.fragment_main, container, false);
R.layout.fragment_main, container, false);
return rootView;
}
}
private class HttpRequestTask extends AsyncTask<Void, Void, Greeting> {
@Override
protected Greeting doInBackground(Void... params) {
try {
final String url = "http://192.168.1.3:8080/greeting";
RestTemplate restTemplate = new RestTemplate();
restTemplate.getMessageConverters().add(
new MappingJackson2HttpMessageConverter());
new MappingJackson2HttpMessageConverter());
Greeting greeting = restTemplate.getForObject(url,
Greeting.class);
Greeting.class);
return greeting;
} catch (Exception e) {
Log.e("MainActivity", e.getMessage(), e);
}
return null;
}
@Override
protected void onPostExecute(Greeting greeting) {
TextView greetingIdText = (TextView) findViewById(R.id.id_value);
TextView greetingContentText = (TextView)
findViewById(R.id.content_value);
findViewById(R.id.content_value);
greetingIdText.setText(greeting.getId());
greetingContentText.setText(greeting.getContent());
}
}
}
Compile và chạy ứng dụng
- Tạo AVD và chạy android client (dùng rest json trả về từ web server) trên android studio
- Kết quả test:
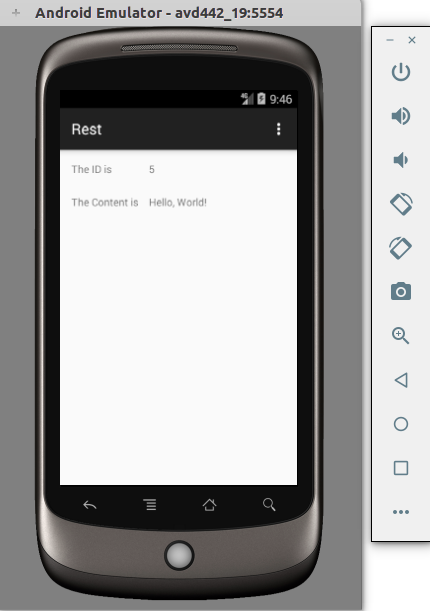
0 comments:
Post a Comment